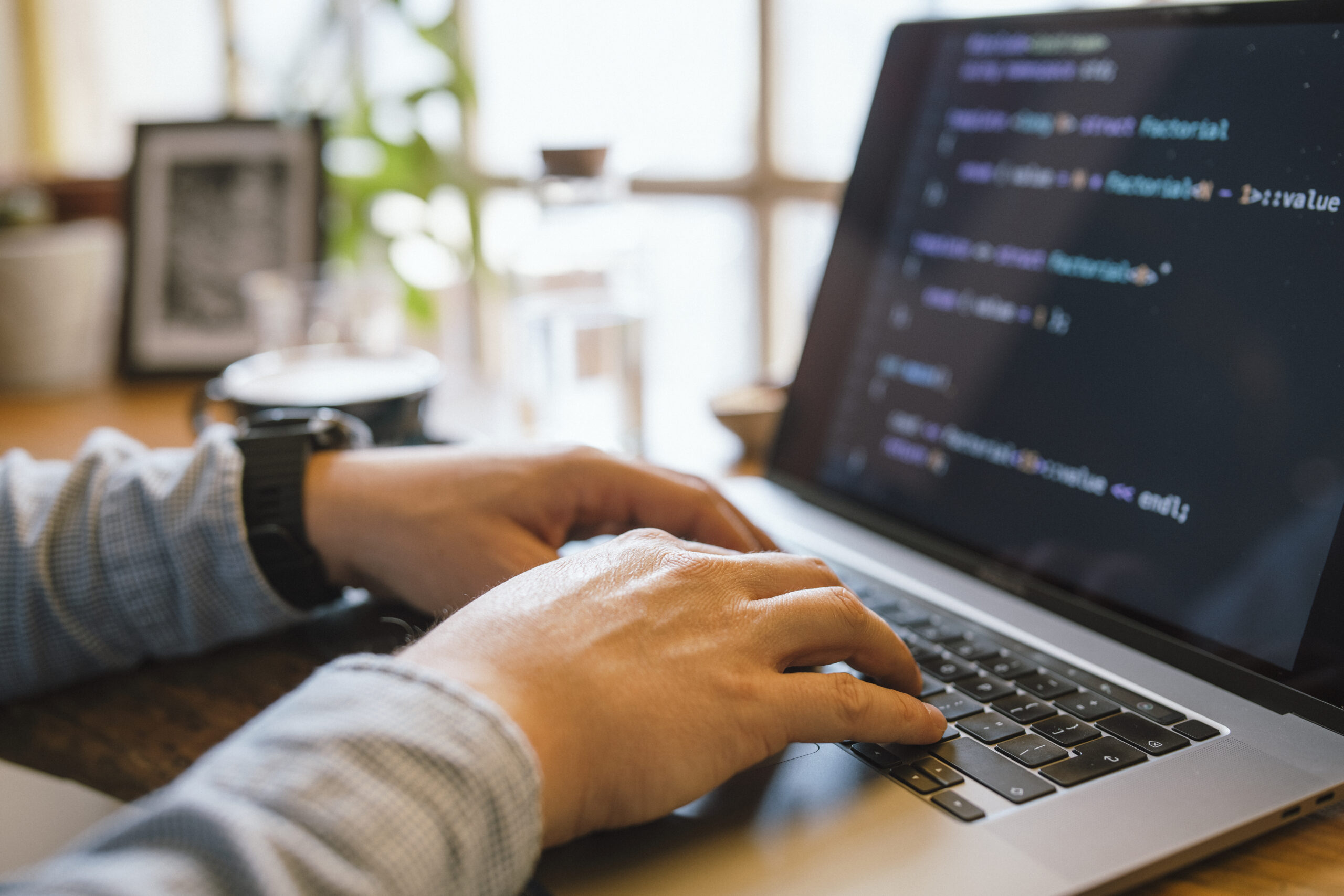
Debugging is Just about the most important — nevertheless normally overlooked — expertise in the developer’s toolkit. It isn't nearly fixing broken code; it’s about comprehension how and why factors go Erroneous, and Understanding to Consider methodically to resolve troubles successfully. Irrespective of whether you are a beginner or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of aggravation and significantly enhance your productiveness. Listed below are numerous techniques to aid developers amount up their debugging sport by me, Gustavo Woltmann.
Master Your Tools
On the list of fastest approaches developers can elevate their debugging capabilities is by mastering the equipment they use daily. Whilst writing code is a person Component of development, recognizing tips on how to communicate with it successfully during execution is Similarly vital. Present day advancement environments come Geared up with effective debugging capabilities — but quite a few builders only scratch the surface area of what these tools can perform.
Consider, such as, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment allow you to established breakpoints, inspect the worth of variables at runtime, stage through code line by line, and in many cases modify code within the fly. When used accurately, they let you observe just how your code behaves in the course of execution, which happens to be priceless for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for front-finish builders. They permit you to inspect the DOM, watch community requests, check out genuine-time general performance metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can change disheartening UI issues into workable tasks.
For backend or technique-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Regulate over working procedures and memory administration. Studying these equipment can have a steeper Finding out curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into comfortable with version Regulate units like Git to know code background, uncover the precise instant bugs were being launched, and isolate problematic alterations.
In the long run, mastering your applications means going beyond default settings and shortcuts — it’s about establishing an personal familiarity with your progress ecosystem so that when issues arise, you’re not dropped at nighttime. The higher you recognize your equipment, the more time you'll be able to devote resolving the particular dilemma as an alternative to fumbling by the method.
Reproduce the trouble
One of the more significant — and infrequently neglected — methods in successful debugging is reproducing the trouble. Prior to jumping in to the code or making guesses, builders will need to make a constant environment or scenario where the bug reliably seems. With no reproducibility, fixing a bug becomes a activity of possibility, generally resulting in squandered time and fragile code improvements.
Step one in reproducing a problem is accumulating as much context as feasible. Talk to inquiries like: What actions triggered The problem? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or error messages? The greater detail you may have, the less difficult it becomes to isolate the precise circumstances under which the bug happens.
When you’ve gathered sufficient facts, make an effort to recreate the condition in your neighborhood atmosphere. This might mean inputting the exact same information, simulating very similar user interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the edge scenarios or condition transitions associated. These tests not merely assistance expose the trouble and also prevent regressions Down the road.
Occasionally, The problem can be environment-certain — it would materialize only on particular functioning systems, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t merely a move — it’s a mindset. It demands persistence, observation, in addition to a methodical approach. But when you can constantly recreate the bug, you are previously midway to repairing it. With a reproducible scenario, You should use your debugging resources much more efficiently, examination likely fixes securely, and communicate more clearly with your team or users. It turns an summary criticism right into a concrete obstacle — Which’s wherever developers thrive.
Study and Comprehend the Error Messages
Error messages tend to be the most valuable clues a developer has when something goes Completely wrong. Rather then observing them as annoying interruptions, developers need to find out to treat mistake messages as immediate communications from your method. They often show you what precisely took place, in which it happened, and in some cases even why it took place — if you know how to interpret them.
Start by looking at the information meticulously and in whole. A lot of developers, specially when below time pressure, look at the primary line and instantly get started generating assumptions. But deeper from the error stack or logs may perhaps lie the genuine root result in. Don’t just duplicate and paste error messages into search engines — examine and comprehend them to start with.
Break the mistake down into areas. Is it a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line quantity? What module or function induced it? These thoughts can guidebook your investigation and point you toward the dependable code.
It’s also useful to be aware of the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable designs, and Mastering to recognize these can dramatically speed up your debugging method.
Some glitches are vague or generic, and in People conditions, it’s essential to examine the context where the error transpired. Test related log entries, input values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings both. These normally precede larger concerns and provide hints about probable bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them the right way turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is Just about the most strong equipment in the developer’s debugging toolkit. When applied proficiently, it offers authentic-time insights into how an software behaves, encouraging you understand what’s happening underneath the hood without having to pause execution or move from the code line by line.
A fantastic logging tactic commences with recognizing what to log and at what amount. Prevalent logging degrees include things like DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic information during development, Facts for normal functions (like profitable commence-ups), WARN for opportunity issues that don’t crack the appliance, ERROR for precise challenges, and Deadly when the procedure can’t keep on.
Prevent flooding your logs with extreme or irrelevant information. Far too much logging can obscure significant messages and slow down your procedure. Center on crucial events, condition improvements, input/output values, and important determination points as part of your code.
Format your log messages Evidently and constantly. Include context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting This system. They’re Specifically important in creation environments where by stepping by means of code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a very well-imagined-out logging tactic, you are able to decrease the time it will require to identify concerns, achieve further visibility into your applications, and improve the In general maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not just a specialized process—it is a method of investigation. To successfully establish and fix bugs, developers need to technique the procedure similar to a detective resolving a secret. This state of mind aids stop working elaborate issues into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Look at the symptoms of the issue: error messages, incorrect output, or efficiency concerns. Similar to a detective surveys a criminal offense scene, acquire as much pertinent facts as you may devoid of leaping to conclusions. Use logs, take a look at situations, and consumer reviews to piece with each other a clear picture of what’s happening.
Subsequent, form hypotheses. Ask you: What can be producing this habits? Have any alterations not too long ago been created towards the codebase? Has this concern occurred right before underneath related conditions? The objective is to slender down opportunities and determine prospective culprits.
Then, test your theories systematically. Seek to recreate the situation in the controlled ecosystem. In case you suspect a specific functionality or part, isolate it and verify if the issue persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the outcomes guide you closer to the reality.
Pay out close awareness to smaller specifics. Bugs often cover within the minimum expected destinations—like a lacking semicolon, an off-by-1 mistake, or even a race condition. Be extensive and patient, resisting the urge to patch The problem without the need of entirely understanding it. Short term fixes may conceal the actual difficulty, just for it to resurface later.
And finally, keep notes on Whatever you tried using and discovered. Equally as detectives log their investigations, documenting your debugging system can conserve time for long run concerns and assistance Other people recognize your reasoning.
By thinking like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more practical at uncovering concealed problems in intricate devices.
Create Exams
Composing assessments is among the simplest ways to boost your debugging capabilities and General advancement effectiveness. Assessments not simply assistance capture bugs early but also serve as a safety net that gives you self-confidence when building variations towards your codebase. A well-tested software is much easier to debug mainly because it allows you to pinpoint exactly exactly where and when a problem occurs.
Begin with unit tests, which concentrate on person functions or modules. These small, isolated tests can immediately expose irrespective of whether a certain bit of logic is Performing as predicted. Each time a examination fails, you right away know exactly where to look, significantly lessening some time put in debugging. Unit tests are Primarily useful for catching regression bugs—challenges that reappear immediately after Earlier getting fixed.
Future, combine integration exams and end-to-conclusion assessments into your workflow. These assist make certain that various aspects of your application work alongside one another efficiently. They’re especially practical for catching bugs that arise in complicated units with a number of components or solutions interacting. If a little something breaks, your exams can show you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Believe critically regarding your code. To test a feature adequately, you'll need to be familiar with its inputs, anticipated outputs, and edge scenarios. This degree of being familiar with By natural means potential customers to better code framework and much less bugs.
When debugging a problem, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails continually, you are able to target correcting the bug and view your examination go when the issue is solved. This approach ensures that the exact same bug doesn’t return Down the road.
In brief, composing assessments turns debugging from the frustrating guessing recreation right into a structured and predictable course of action—helping you catch additional bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s easy to become immersed in the issue—watching your display screen for hrs, hoping Alternative after Remedy. But The get more info most underrated debugging instruments is solely stepping absent. Getting breaks can help you reset your intellect, cut down frustration, and often see The difficulty from the new standpoint.
If you're far too close to the code for too long, cognitive exhaustion sets in. You might start overlooking noticeable faults or misreading code that you choose to wrote just several hours before. In this particular condition, your brain gets to be much less productive at difficulty-solving. A brief wander, a coffee break, or even switching to another undertaking for ten–15 minutes can refresh your concentrate. Many builders report obtaining the foundation of a difficulty after they've taken time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially throughout longer debugging classes. Sitting before a monitor, mentally caught, is not just unproductive but will also draining. Stepping away enables you to return with renewed Electrical power plus a clearer state of mind. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you before.
When you’re stuck, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a 5–ten minute crack. Use that time to maneuver around, stretch, or do something unrelated to code. It might experience counterintuitive, Primarily below limited deadlines, however it essentially leads to speedier and more effective debugging Eventually.
To put it briefly, using breaks will not be a sign of weak point—it’s a wise strategy. It provides your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and relaxation is part of fixing it.
Study From Each Bug
Each and every bug you face is a lot more than just A brief setback—It is really an opportunity to develop being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural concern, each can instruct you a little something beneficial should you make time to replicate and review what went wrong.
Start off by inquiring on your own a handful of vital questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with far better procedures like unit screening, code evaluations, or logging? The solutions usually reveal blind spots with your workflow or comprehension and make it easier to Make more robust coding practices relocating forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and Whatever you uncovered. With time, you’ll begin to see patterns—recurring issues or common issues—you could proactively prevent.
In crew environments, sharing Everything you've discovered from the bug using your peers can be Primarily highly effective. No matter whether it’s through a Slack information, a short write-up, or a quick know-how-sharing session, supporting Other people steer clear of the very same concern boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. In fact, several of the best builders are not the ones who generate excellent code, but those who continually master from their blunders.
Eventually, Each and every bug you take care of adds a whole new layer to your ability established. So next time you squash a bug, take a minute to reflect—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and tolerance — nevertheless the payoff is big. It will make you a more effective, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become superior at what you do.